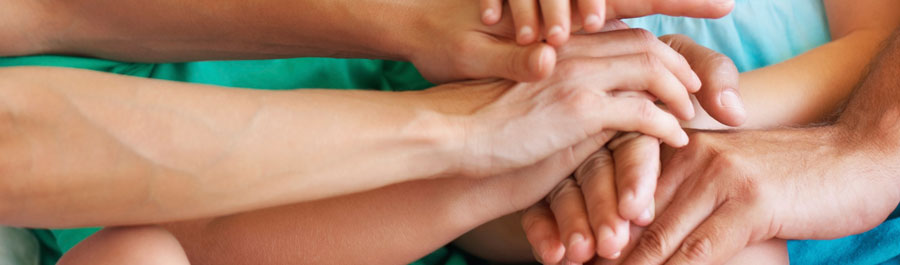
Microchip PIC24 Assembly Language Programming
Duration: 5 Days
Course Background
A knowledge of assembly language programming is a key skill for small embedded systems application developers. Not only does an understanding of the microcontroller instruction set help with debugging, it can be used to optimise code in resource constrained systems. It is also an important skill to have when implementing applications that are a mix of assembler and C code and when implementing low level device drivers. The Microchip 16 bit Microcontroller family has a richer instruction set than that of the 8 bit family. There is however a considerable overlap. The dsPIC family differs from the PIC24 family in having extra hardware concerned with DSP (Digital Signal Processing) and extra instructions for this very purpose. In this course the emphasis will be on the PIC24 instruction set and also the use of the same instructions in dsPIC processors. The dsPIC DSP course will cover the DSP related instructions found in the dsPIC family of microcontrollers
This course will cover techniques for modular programming in assembler and the structured use of macros. It should also be of use to those teaching or developing computer science courses who will be delivering a module on more advanced microprocessor architectures, instruction sets and programming at the level of instruction sets.
Laboratory Workshops
- Program object code into a target PIC24 /dsPIC microcontroller using MPLABX and ICD3
- Create, build and debug new assembly language projects using MPLABX and ICD3
- Use the MPLAB X Simulator to simulate and debug code prior to programming it into a target device
- Understand the components of an assembly source file
- Setting the PIC24 and dsPIC configuration options appropriately for the target system
- Use digital I/O ports to interact with the outside world
- Manipulating data memory using direct and indirect addressing
- Create time delays using software loops and hardware timers
- Writing code for interrupt handling and stack manipulation
- Take advantage of interrupts to handle events in the background
- Jump and call routines across pages in program memory
- Use of PC-relative addressing to implement look-up tables
- Implementing robust design techniques to protect against malfunctions
Course Prerequisites and Target Audience
Basic knowledge of programming and working with PC tools is assumed. Attendees are also expected to have a working knowledge of number systems decimal, octal, hexadecimal and binary and basic logic operations such as AND, OR, Exclusive OR and NOT. An appreciation of basic approaches to structured programming would also be helpful.
Course Outline
- Market profile
- product range
- key advantages and support network
- Microchip development software: MPLABX and associated tools
- Microchip In-Circuit Debugger (ICD3) and In-Circuit Emulator (ICE) hardware
- Device programmers, and the design option of In-Circuit Serial Programming (ICSP)
- Demonstration boards and kits
- Third-party development tools
- The difference between a microcntroller and a microprocessor
- Harvard versus Von Neumann architecture
- Organisation of program and data memory
- RISC architecture with multiple working registers
- Op-codes and addressing modes: immediate, direct and indirect
- On-chip peripherals and interrupts
- Classes of operations performed by op-codes
- The System Clock
- Resets and Reset circuits
- Differences in instruction sets and memory architecture between PIC24 and dsPIC microcontrollers
- Understanding microcontroller pinouts and schematic diagrams
- The PIC24 Microcontroller Instruction Sets
- Data Transfer Instructions
- Arithmetic Logic Instructions
- Bit-oriented Instructions
- Program Control Instructions
- Other Instructions
- Understanding PIC24 Microcontroller Addressing Modes
- Direct addressing
- Register Direct Addressing
- Register Indirect Addressing
- Indexed Addressing
- EDS - Extended Data Space Addressing
- Special Function Registers and Ports
- The need for Special Function Registers
- SR - ALU STATUS Register
- CORCON - CPU Control Register
- ICN Register
- TIMER Register
- INPUT Capture Register
- Output Compare Register
- SYSTEM Register
- NVM Register
- PMD - Peripheral Module Disable Register an PIC24 assembler language application
- Brief Introduction to Structured Analysis and Design using Ward-Mellor / Hatley Pirbhai / Jackson methodologies
- TRIS Register Maps
- PORT RegisterMaps
- ANSEL Register
- RCON - Reset Control Register
- Interrupt Vector Table
- Interrupt Related SFRs - INTCON1, INTCON2, IFS0 - IFS6, IEC0 - IEC6, IPC0 - IPC25, INTTREG
- Overview of Peripheral Associated Special Function Registers
- Designing and implementing programs and applications
- Requirements analysis
- Describing algorithms using pseudo code (structured English)
- Describing algorithms using flow charts
- Basic template for an PIC24 assembler language application
- Brief Introduction to Structured Analysis and Design using Ward-Mellor / Hatley Pirbhai / Jackson methodologies
- Context Diagram
- Flow diagrams
- Control diagrams
- Finite State Machines
- Function mini-specs and pseudo code
- Macros
- Macros as pattern substitution
- Macros with parameters
- Macros and subroutines compared
- Macros with parameters
- Common Macro based patterns and idioms
- Interrupts
- The interrupt handling architecture of the PIC24
- Interrupts and Event Driven Systems
- Synchronous vs. Asynchronous Event Handling
- Template for implementing an interrupt handler
- Effective use of interrupt priorities in the PIC24
- Digital Inputs and Outputs
- Programming Digital Outputs
- Programming Digital Inputs
- Using Polling to detect input changes
- Using Edge Triggered Interrupts to detect input changes
- Debouncing
- Programming a matrix keypad
- Timers
- How timers work
- Timeout events and timeout handlers
- Capture Compare
- Pulse width modulation
- Timers for generating periodic events
- Timers and multi-tasking
- Analog Inputs
- Understanding Analog to Digital Conversion (ADC)
- ADC architectures
- Program templates for working with analog inputs
- Foundations of signal processing
- Use analog inputs to control and monitor
- Serial buses and Protocols
- RS232
- I2C
- SPI
- Introduction to multi-tasking