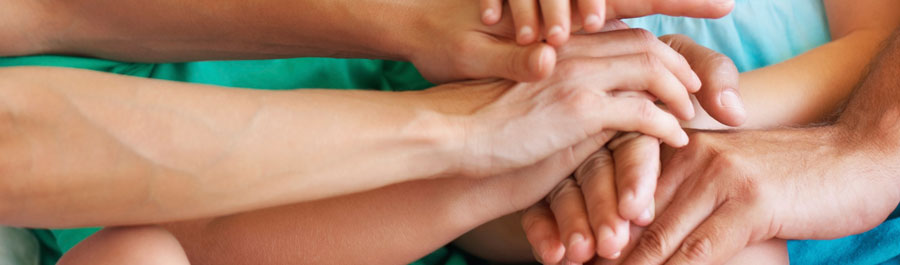
ARM Cortex-M Assembly Language Programming
Duration: 5 Days
Course Background
A knowledge of assembly language programming is a key skill for small embedded systems application developers. Not only does an understanding of the microcontroller instruction set help with debugging, it can be used to optimise code in resource constrained systems. It is also an important skill to have when implementing applications that are a mix of assembler and C code and when implementing low level device drivers.
This course will cover techniques for modular programming in assembler and the structured use of macros. It should also be of use to those teaching or developing computer science courses who will be delivering a module on more advanced microprocessor architectures, instruction sets and programming at the level of instruction sets.
Laboratory Workshops
- Program object code into a target ARM CortexM processor using JTAG from the IDE
- Create, build and debug new assembly language projects using the IDE
- Using an ARM CortexM Simulator to simulate and debug code prior to programming it into a target device
- Understand the components of an assembly source file
- Implement Startup Code for the Cortex-M
- Use digital I/O ports to interact with the outside world
- Manipulate data memory using direct and indirect addressing
- Create time delays using software loops and hardware timers
- Writing code for interrupt handling and stack manipulation
- Take advantage of interrupts to handle events in the background
- Jump and call routines
- Use of PC-relative addressing to implement look-up tables
- Implementing robust design techniques to protect against malfunctions
Course Prerequisites and Target Audience
Basic knowledge of programming and working with PC tools is assumed. Attendees are also expected to have a working knowledge of number systems decimal, octal, hexadecimal and binary and basic logic operations such as AND, OR, Exclusive OR and NOT. An appreciation of basic approaches to structured programming would also be helpful.
Course Outline
- Strategy and Thinking behind the Cortex-M
- Varieties of Cortex-M Core Architectures
- Varieties of Cortex-M Processors
- Keil IDE and Compiler
- IAR Embedded Workbench IDE and Compiler
- Atmel Studio
- TI Code Composer Studio
- TrueStudio - Atollic
- GCC for ARM Embedded Processors and Rowley CrossWorks for ARM
- The difference between a microcntroller and a microprocessor
- Harvard versus Von Neumann architecture
- Organisation of program and data memory
- RISC architecture with multiple working registers
- Special registers
- Memory Map
- Bit Banding
- Overviw of the ARM Cortex-M Instruction Sets
- 16 bit Thumb and 32 bit Thumb2 Instructions
- Operating Modes
- The System Clock
- Resets and Reset circuits
- NVIC - Nested Vector Interrupt Controller
- Unified Assembly Language (UAL)
- 32bit Instruction Encoding
- 16bit Instruction Encoding
- Application Program Status Register (APSR)
- Conditional Execution
- Instruction Width Selection
- Instruction Set Details
- Data Processing Instructions
- Data Transfer Instructions
- Block Transfer Instructions
- Branching Instructions
- Multiply Instructions
- Software Interrupt Instructions
- Cortex-M processor architecture and Instruction Set Details
- Logical ARM Cortex-M Memory Map Model
- Memory Map Regions - Code, SRAM, Peripheral, External RAM, External Device, Private Peripheral Bus, Vendor Specific Memory
- Offset Addressing
- Pre-Indexed Addressing
- Post-Indexed Addressing
- Offset options
- IO-Ports
- DMA Controller
- EXTI - External Interrupt
- ADC
- Multi ADC
- DAC
- DCMI - Digital Camera Interface
- Control Timers
- PWM - Pulse Width Modulation
- Capture Compare
- RS232
- SPI
- I2C
- Assembly Directives
- Macros
- Structured Programming Using Macros
- Functions and function calling
- ARM Procedure Call Standard (AAPCS)
- Linker
- Implementing an application as a collection of modules
- External Programming
- In-System Programming (ISP)
- In Application Programming (IAP)
- Survey of various Flash Utilities
- Requirements analysis
- Describing algorithms using pseudo code (structured English)
- Describing algorithms using flow charts
- Basic template for an PIC32 assembler language application
- Brief Introduction to Structured Analysis and Design using Ward-Mellor / Hatley Pirbhai / Jackson methodologies
- Context Diagram
- Flow diagrams
- Control diagrams
- Finite State Machines
- Function mini-specs and pseudo code
- Macros as pattern substitution
- Macros with parameters
- Macros and subroutines compared
- Macros with parameters
- Common Macro based patterns and idioms
- The interrupt handling architecture of the ARM Cortex-M
- Interrupts and Event Driven Systems
- Synchronous vs. Asynchronous Event Handling
- Template for implementing an interrupt handler
- Effective use of interrupt priorities in the ARM Cortex-M
- Programming Digital Outputs
- Programming Digital Inputs
- Using Polling to detect input changes
- Using Edge Triggered Interrupts to detect input changes
- Debouncing
- Programming a matrix keypad
- How timers work
- Timeout events and timeout handlers
- Capture Compare
- Pulse width modulation
- Timers for generating periodic events
- Timers and multi-tasking
- Understanding Analog to Digital Conversion (ADC)
- ADC architectures
- Program templates for working with analog inputs
- Foundations of signal processing
- Use analog inputs to control and monitor
- RS232
- I2C
- SPI