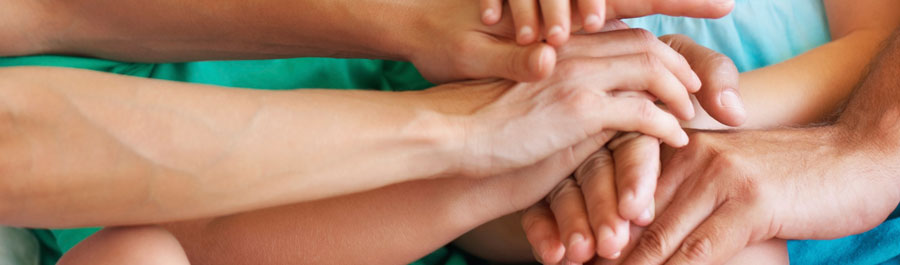
Linux Embedded System C++ Programming
Duration: 5 Days
Course Background
This course is designed for experienced C programmers with some experience of either embedded system or Win32 API or POSIX API programming, but who have little experience of either C++ programming, or object oriented programming or both. As well as providing a fast paced introduction to the C++ programming language the course also explores topics that are paraticularly relevant to embedded systems developers such as C++11 multi-threading, C++ Stream Libraries and C++ Network and Asynchronous IO programming. The course will also overview C++ approaches to user space GPIO, RS232 serial and I2C/SPI serial C++ programming.
Course Overview
This intensive, fast paced course enables experienced C programmers to begin writing embedded C++ software. It covers all the commonly used features of the C++ language and provides insights into the strengths and limitations of these features in the context of implementing embedded applications. Additionally, the course discusses the implemention of C++ class wrappers around C-based operating system APIs and TCP/IP socket APIs and explores C++ techniques for working with GPIO and with serial protocols such as RS232, SPI and I2C.
Practical work uses the GNU C++ compiler running on a Linux workstation, as well as cross-compiling projects to run on embedded Linux platforms. The platforms used on the course will be the RaspberryPi and the BeagleBone Black. Projects are built using both the traditional command-line-and-Makefile approach, and also using an Eclipse based C++ Integrated Development Environment. Applications are run on a PC running one of the standard Linux distributions, and also on an ARM board running embedded Linux.
Course Benefits
Migrating from C to C++ is challenging task if the true benefits of using C++ are to be realised. Unless careful thought is given to Analysis and Design, the resulting code may be little more than C code with C++ wrappers, with little or no gain in productivity through using an object-oriented language.
The real benefits of C++ come from:
- Expertise in object oriented analysis and design
- An understanding of object oriented programming patterns and idioms
- The ability to identify and use existing C++ frameworks, or to design and implement new frameworks from scratch
- Experience with the C++ STL (Standard Template Library) and the Boost C++ libraries
On completing this course you will
- Have a sound understanding of UML Notation to specify C++ applications and an ability to think and work with objects as the underlying abstraction
- Understand polymorphism and be able to identify situations where the use of polymorphism in an embedded system environment is appropriate
- Be able to make the best use of constructors and destructors, delete and new operators and understand key idioms and patterns such as reference counting, copy on modify, and the Singleton.
- Be able to write C++ code that can access embedded system hardware
- Understand the advantages and disadvantages of wrapping C operating system API calls in C++ classes
- Understand C++ exception handling and the C++ RTTI (Run Time Type Identification) mechanism, and identify situations where their use is appropriate (or not)
- Be aware of object oriented patterns and their uses
- Be aware of framework oriented approaches and their uses
- Appreciate how to realise dynamic data structures and their associated algorithms in C++
- Understand the principles underlying templates and the STL, and decide where the use of templates and the STL is appropriate
- Be aware of C++ techniques for implementing small memory systems
- Be able to build applications that combine C++, C and assembler and that reuse existing C code and libraries
- Understand basic principles and strategies for wrapping operating system API functions in C++ clases - e.g. for multi-threading and inter-process communication
- Understand the design underlying the C++ IOSTREAM classes, where to use them in embedded applications, and where to use low level I/O in the C standard library instead.
Course Contents
- Brief History of C++
- C++ as an extension of C
- C++ as an evolving language and the development of the ANSI C++ standard
- Possible memory and performance costs of using C++ in embedded applications
- Overview of new features in C++ 11
- Review of the C in C++
- Base data types (numeric char, integer and floating point types)
- Pointers and arrays
- Function prototypes and function pointers
- structs , pointers to structs and dynamic data structures
- typedefs, enums and macros
- I/O in C
- Replacing C based I/O (printf, scanf etc) with C++ based I/O
- Functions in C++
- Function overloading
- Name mangling / decorating
- Mixing C and C++ code modules (use of extern C)
- Operators and operator overloading in C++
- Key Object Orientated Concept - Classes and Instances
- Member variables and member functions (private, public, protected)
- Constructors and destructors
- Default constructors and default destructors
- Initialiser lists
- Derived classes
- Arrays of classes
- UML class diagram notation
- More advanced aspects of working with classes and instances
- Copy constructors
- Deep vs. shallow copying
- Virtual functions and virtual classes (compile time vs. run time binding)
- Implementing dynamic data structures using classes (linked lists, binary trees, etc)
- Friend classes and friend functions
- Static member variables and static member functions
- Templates and the Standard Template Library
- A more detailed look at input and output in C++
- The C++ iostream classes
- Overloading the insertion and extraction operators for use with user defined classes
- Reading and writing files
- Basics of object and data persistence
- Dynamic memory management
- The new and delete operators
- Overloading the new and delete operators
- Application specific memory management
- Exception Handling
- The concept and structure of exceptions and exception handling
- Throwing an exception
- The try block
- Catching an exception
- Re-throwing exceptions
- Catch-all handlers
- Exception specifications
- Exception handling implemention mechanisms
- Appropriateness of exception handling in embedded applications
- Alternatives to the C++ exception handling mechanism
- Templates and their uses
- Data parameterised collections of classes and functions
- How C++ compilers handle templates
- The C++ Standard Template Library - a brief overview
- The Boost C++ libraries - a brief overview
- Namespaces - an extra level of scoping
- usefulness of namespaces in large projects and when re-using code
- Mixed C and C++ applications and extern C
- Mixed C++, C and assembler applications
- Calling assembler from C
- Calling assembler from C++
- Structured use of the Posix API in C++
- Task and thread creation and management
- Semaphores, mutexes and conditional variables
- Memory mapping and inter-process communication
- Sockets API in C++ - TCP/IP Sockets libraries
- File IO
- Accessing peripherals and devices from user space
- Patterns and idioms for accessing hardware from C++ in User Space Linux applications
- Patterns and idioms for bit manipulation in C++
- Memory mapping and access to memory mapped device registers from user space
- Polling techniques for synchronous I/O
- Working with I2C and SPI devices
- Working with RS232 and RS485
- GCC Compiler Specific Extensions to C++
- Accessing Special Function Registers (SFRs)
- #pragma s
- Compiler-specific non-ANSI C++ extensions
