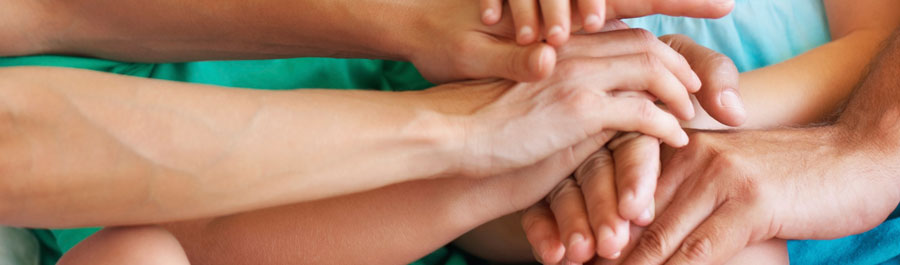
Linux Kernel Internals and Device Driver Programming
Duration: 5 Days
Course Background
Developers building embedded solutions using Linux often need to make kernel-level modifications, or to write drivers for custom hardware. This advanced programming course provides an intensive overview of the Linux 3.x kernel, kernel level programming and device driver theory and implementation, as well as kernel configuration and compilation. Code will be developed and tested on both a Debian 3.x kernel running on a PC Platform and a suitable embedded Linux distribution running on either a RaspberryPi or BeagleBone Black platform. [Note: the course can be tailored to use alternative target boards if required]. Linux is quite a complex operating system and mastery depends on understanding the underlying patterns and abstractions involved in developing various elements of the kernel. The Linux 3.x kernel is simply a continuation of the 2.6 kernel. This course will be based on a reasonably uptodate 3.x Kernel that can be considered to be sufficiently stable for use in real world embedded systems applications. The kernel internals aspects of the course will concentrate on mastering the architectural details of the various kernel subsystems as well as understanding the various scheduler configurations, kernel space multi-tasking and user-space multi-tasking. In addition the course will cover memory mapping and memory management, /dev, /proc and /sys filesystems and their uses as well as reviewing the various device classes and drivers that are part of the Linux kernel. Linux system build and configuration will be covered as well as the building of actual distributions.
Intended Audience
Attendees are expected to be proficient C programmers and have a good working knowledge of shell programming and the use of Linux tools and utilities.
Course Overview
Course Benefits
You will gain a comprehensive understanding of Linux Kernel building, kernel and device driver programming, and experience of device drivers for components and protocols often found in embedded systems such as I2C, SPI, Bluetooth and USB. You'll consolidate your knowledge with practical labs, including:
- Investigating process creation and management in the Linux kernel
- Investigating inter-process communication mechanisms
- Implementing and testing a loadable module
- Building a character device driver as a loadable module
- Building and testing an I2C network interface driver
Course Contents
- Linux Background
- The evolution of Linux
- Linux and the POSIX API
- Understanding how the Linux Kernel is split up
- The evolution of Linux from 1.x to 2.x to 3.x
- Linux Internals - the key parts
- Memory addressing - segmentation and paging
- Process creation, process switching, process destruction
- Interrupts and Exception Handling
- Time, timing and timer interrupts
- Memory management and the process address space
- System calls, signals and the POSIX API
- I/O Devices
- File systems - VFS, EXT2, Flash File Systems
- Inter-process communication
- Program loading and execution
- Modules
- Loadable modules and insmod
- Security issues with loadable modules
- Applications compared to kernel modules
- User space, kernel space
- Compiling and loading a module
- Initialisation, shutdown and error handling by a module
- Usage counting and module unloading
- How a module acquires and accesses system resources (I/O Ports, I/O memory)
- Automatic and manual configuration
- User space drivers
- Character Drivers
- Major and minor device numbers
- Dynamic allocation of major numbers
- File operations - the file_operations structure and the file structure
- Case study - Rubini and Corbet's scull device
- Getting applications to use new devices
- Device drivers
- Device driver testing and debugging
- Using ioctl commands
- Blocking vs. non-blocking I/O
- Controlling access to a device
- Time and the Linux Kernel
- Timer interrupts and kernel time
- Kernel time and the jiffies variable
- Task queues
- Understanding kernel timers
- Tasklets and deferred work
- Memory management - basics
- kmalloc
- Lookaside caches
- vmalloc
- Boot time memory allocation
- Hardware management and usage - basics
- I/O Ports and I/O Memory
- Interrupts and Interrupt handling
- The /proc interface
- Circular buffers
- Spinlocks
- Race conditions
- Block drivers
- Registering
- Handling requests - and data transfer
- Queuing and block drivers
- The ioctl command and block drivers
- Case studies
- USB device drivers
- I2C and SPI device drivers
- CAN device drivers
- Bluetooth device drivers
- Network device drivers
- Overview of the Linux TCP/IP Protocol stack
- TCP/IP over Ethernet
- A/D and D/A for control applications
- /proc and syfs - Advanced Topics
- Exploring the /proc file system
- Implementing /proc capable device drivers
- Understanding the purpose and architecture of sysfs
- Examining USB and I2C device driver sysfs related details