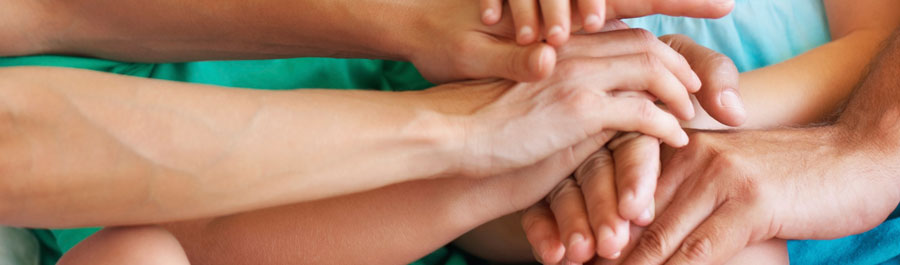
Course 8051ASM - 8051 Assembly Language Programming
Duration: 5 Days
Course Background
A knowledge of assembly language programming is a key skill for small embedded systems application developers. Not only does an understanding of the microcontroller instruction set help with debugging, it can be used to optimise code in resource constrained systems. It is also an important skill to have when implementing applications that are a mix of assembler and C code and when implementing low level device drivers.
Although the course can be tailored to a variety of 8 bit microcontrollers the standard version of the course is taught using either a Silabs 8051 based microcontroller together with the Keil IDE, or an Atmel 8051 based microcontroller with the Atmel AT89 Developer Studio and SDCC (Small Device C Compiler).
Course Prerequisites and Target Audience
Basic knowledge of programming and working with PC tools is assumed. Attendees are also expected to have a working knowledge of number systems decimal, octal, hexadecimal and binary and basic logic operations such as AND, OR, Exclusive OR and NOT. An appreciation of basic approaches to structured programming would also be helpful.
Course Outline
- Overview of the 8051 processor architecture
- The difference between a microcntroller and a microprocessor
- 8051 memory organisation - program memory and data memory
- The System Clock
- Resets and Reset circuits
- Understanding microcontroller pinouts and schematic diagrams
- Understanding 8051 Addressing Modes
- Direct addressing
- Register Direct Addressing
- Register Indirect Addressing
- Indexed Addressing
- Special Function Registers and Ports
- Register A/Accumulator
- Register B
- Port Registers
- Stack Pointer
- Power Management Register (PCON)
- Processor Status Word (PSW)
- Enhancements to the classical 8051 processor architecture in modern 8051 microcontrollers
- Exploring the Instruction Sets of the 8051
- Instructions for data transfer/ data move
- Instructions for arithmetic operations
- Instructions for branching a program
- Instruction for creating subroutines
- Instructions for logical operations
- Instructions for boolean operations
- Special purpose instructions
- Designing and implementing programs and applications
- Requirements analysis
- Describing algorithms using pseudo code (structure English)
- Describing algorithms using flow charts
- Basic template for an 8051 assembler language application
- Introduction to Structured Analysis and Design using Ward-Mellor / Hatley Pirbhai / Jackson methodologies
- Context Diagram
- Flow diagrams
- Control diagrams
- Finite State Machines
- Function mini-specs and pseudo code
- Macros
- Macros as pattern substitution
- Macros with parameters
- Macros and subroutines compared
- Macros with parameters
- Common Macro based patterns and idioms
- Interrupts
- The interrupt handling architecture of the 8051
- Interrupts and Event Driven Systems
- Synchronous vs. Asynchronous Event Handling
- Template for implementing an interrupt handler
- Digital Inputs and Outputs
- Programming Digital Outputs
- Programming Digital Inputs
- Using Polling to detect input changes
- Using Edge Triggered Interrupts to detect input changes
- Debouncing
- Programming a matrix keypad
- Timers
- How timers work
- Timeout events and timeout handlers
- Capture Compare
- Pulse width modulation
- Timers for generating periodic events
- Timers and multi-tasking
- Analog Inputs
- Understanding Analog to Digital Conversion (ADC)
- ADC architectures
- Program templates for working with analog inputs
- Foundations of signal processing
- Use analog inputs to control and monitor
- Serial buses and Protocols
- RS232
- I2C
- SPI
- Introduction to multi-tasking